Have you ever been frustrated by messy, unorganized data? Imagine trying to answer a question, but your data is riddled with errors, inconsistencies, and missing values. This is a common scenario data scientists face, and it’s where the magic of data preprocessing comes in. Data preprocessing is the essential step of cleaning and transforming your data into a format that’s ready for analysis and modeling. And the best part? Python, with its powerful libraries and intuitive syntax, makes this process a breeze!
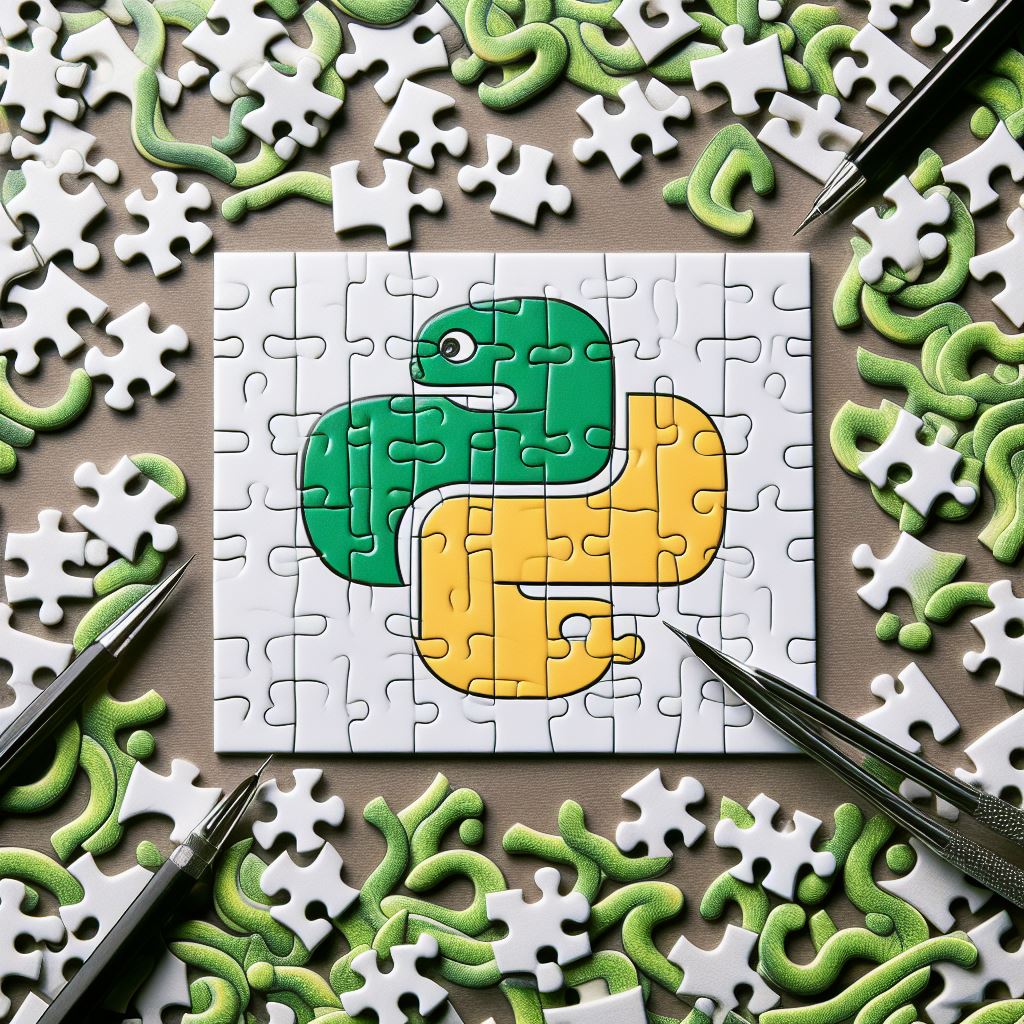
Image: hataftech.com
This guide dives deep into hands-on data preprocessing in Python. Learn how to tackle common data cleaning challenges like handling missing values, transforming data types, and identifying outliers. We’ll cover crucial techniques like feature scaling, encoding categorical variables, and more. By the end, you’ll equip yourself to transform raw data into a polished dataset ready to unlock valuable insights.
Understanding the Importance of Data Preprocessing
Data preprocessing is not just about tidying up your dataset; it’s about ensuring the quality and relevance of your data for successful analysis. Here’s why it’s crucial:
- Improved Model Accuracy: Clean and consistent data leads to more accurate predictions and insights from your machine learning models. Garbage in, garbage out!
- Enhanced Feature Engineering: Data preprocessing enables you to create new features by combining existing ones, paving the way for more effective model training.
- Faster Algorithm Convergence: Preprocessing techniques like feature scaling can optimize the speed and efficiency of your machine learning algorithms.
- Reduced Bias: Handling outliers and imbalances in your data during preprocessing helps to mitigate bias and create fairer models.
Essential Libraries for Data Preprocessing in Python
Python boasts a rich ecosystem of libraries tailored for data preprocessing. The following are among the most popular and widely used:
- NumPy: The foundation of scientific computing in Python, NumPy provides efficient array manipulation, mathematical operations, and random number generation.
- Pandas: A data analysis powerhouse, Pandas offers data structures like DataFrames and Series, allowing you to read, clean, transform, and analyze data with ease.
- Scikit-learn: The go-to library for machine learning in Python, it includes a comprehensive suite of preprocessing tools for handling missing values, scaling features, encoding categorical variables, and more.
- Matplotlib & Seaborn: These visualization libraries help you explore and understand your data visually, identifying patterns and anomalies that could guide your preprocessing decisions.
Hands-On Data Preprocessing Techniques: A Step-by-Step Guide
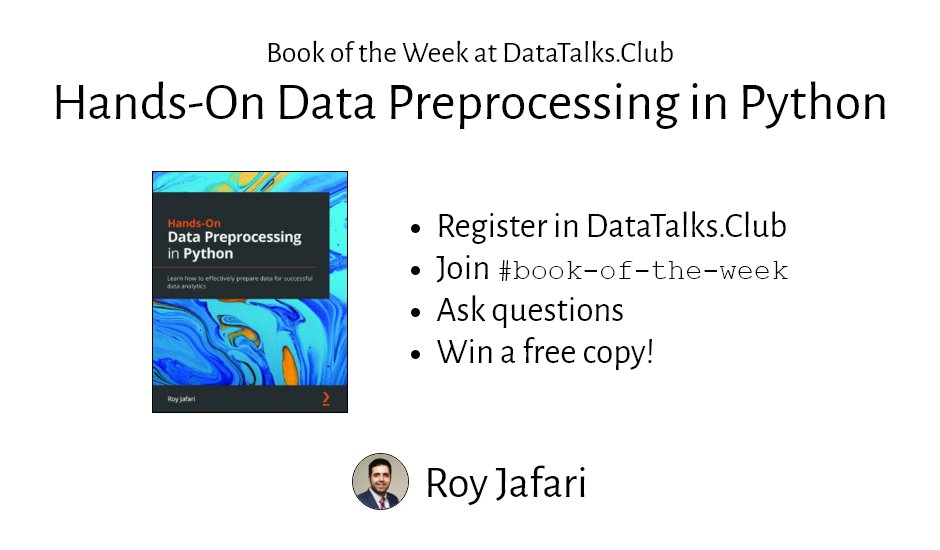
Image: datatalks.club
1. Handling Missing Values
Missing values are a common problem in real-world datasets. Here’s how to address them in Python:
1. Detection:
- Use
df.isnull().sum()
to count missing values in each column of your Pandas DataFrame. - Visualize missing data using a heatmap to identify potential patterns.
2. Imputation:
- Mean Imputation: Replace missing values with the average of the non-missing values in the same column. Useful for numerical features.
- Median Imputation: Similar to mean imputation but uses the median instead. Robust to outliers.
- Mode Imputation: Replace missing values with the most frequent value in the column. Suitable for categorical features.
- K-Nearest Neighbors Imputation: Uses the values of neighboring data points to impute missing values. Effective when data has more complex relationships.
Example:
import pandas as pd
from sklearn.impute import SimpleImputer
# Load your data
df = pd.read_csv('your_data.csv')
# Create an imputer object
imputer = SimpleImputer(strategy='mean')
# Fit the imputer to your data
imputer.fit(df[['age', 'income']])
# Transform the data to fill missing values
df[['age', 'income']] = imputer.transform(df[['age', 'income']])
2. Transforming Data Types
Data types play a crucial role in how your data is interpreted and processed. Ensure that they align with your analysis goals.
1. Data Type Conversion:
- Use
astype()
to convert data types explicitly. For example,df['age'] = df['age'].astype(float)
converts the ‘age’ column to floating-point numbers. - Utilize
to_datetime()
to convert strings to datetime objects for temporal data.
2. Handling Categorical Variables:
- One-Hot Encoding: Creates binary columns for each category, indicating the presence or absence of that category. Use
pd.get_dummies()
for one-hot encoding. - Label Encoding: Assigns numerical labels to categorical values. Use
sklearn.preprocessing.LabelEncoder()
for label encoding.
Example:
import pandas as pd
from sklearn.preprocessing import LabelEncoder
# Load your data
df = pd.read_csv('your_data.csv')
# Create a LabelEncoder object
le = LabelEncoder()
# Fit and transform the 'gender' column
df['gender'] = le.fit_transform(df['gender'])
3. Feature Scaling
Feature scaling is essential for algorithms that are sensitive to feature scales, like k-Nearest Neighbors, Support Vector Machines, and neural networks. It ensures that features contribute equally to model training.
- Min-Max Scaling: Rescales features to a specified range, typically [0, 1]. Use
sklearn.preprocessing.MinMaxScaler()
. - Standard Scaling (Z-score): Standardizes features by subtracting the mean and dividing by the standard deviation. Use
sklearn.preprocessing.StandardScaler()
.
Example:
from sklearn.preprocessing import MinMaxScaler
# Create a MinMaxScaler object
scaler = MinMaxScaler()
# Fit the scaler to your data
scaler.fit(df[['age', 'income']])
# Transform the data
df[['age', 'income']] = scaler.transform(df[['age', 'income']])
4. Handling Outliers
Outliers are extreme values that deviate significantly from the rest of the data. They can skew your analysis and model performance.
- Visualization: Use box plots or scatter plots to identify outliers visually.
- Statistical Methods: Use interquartile range (IQR) or z-score to detect outliers based on their position within the data distribution.
- Removal: Remove outliers if they are clearly errors or don’t represent the true distribution of the data.
- Transformation: Transform features with outliers using techniques like log transformation or Box-Cox transformation to reduce their influence.
Example:
import numpy as np
# Calculate the IQR
Q1 = np.percentile(df['income'], 25)
Q3 = np.percentile(df['income'], 75)
IQR = Q3 - Q1
# Define outlier thresholds
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
# Remove outliers
df = df[(df['income'] >= lower_bound) & (df['income'] <= upper_bound)]
5. Feature Engineering
Feature engineering is the art of creating new features from existing ones to enhance model performance. It involves extracting relevant information and patterns from your data.
- Interactions: Combine existing features to capture non-linear relationships. For example, create a new feature ‘age * income’ to assess the combined effect of age and income.
- Polynomial Features: Add polynomial terms (e.g., squares, cubes) of existing features to model non-linear relationships.
- Binning: Group continuous variables into discrete bins for easier interpretation and analysis.
- Domain Knowledge: Leverage your understanding of the problem domain to create features that are meaningful and potentially predictive.
Example:
from sklearn.preprocessing import PolynomialFeatures
# Create a PolynomialFeatures object
poly = PolynomialFeatures(degree=2)
# Fit and transform the data
df_poly = poly.fit_transform(df[['age', 'income']])
Benefits of Using Python for Data Preprocessing
Python’s popularity in data science stems from its rich ecosystem of libraries, ease of use, and flexibility. Its benefits for data preprocessing include:
- Comprehensive Libraries: Python offers a wide range of specialized libraries for data manipulation, cleaning, and transformation.
- Intuitive Syntax: Python’s code is highly readable and easy to understand, making it beginner-friendly.
- Scalability: Python allows you to work with large datasets efficiently, thanks to libraries like Dask and PySpark.
- Open Source and Free: Python is a free and open-source language, making it accessible to everyone.
- Active Community: Python enjoys a vibrant and supportive community, providing abundant resources and knowledge sharing.
Hands On Data Preprocessing In Python Pdf
Conclusion:
Data preprocessing is a fundamental step in any data science project. Python, with its powerful libraries and user-friendly syntax, empowers you to handle messy data effectively, transforming it into a valuable asset for your analysis. By mastering techniques like handling missing values, transforming data types, scaling features, and identifying outliers, you’ll ensure that your data is ready to reveal valuable insights and drive informed decision-making.
So, embark on your data preprocessing journey with Python! Explore the countless resources available online, experiment with different techniques, and build confidence in your ability to clean and prepare data for meaningful analysis. Happy preprocessing!