Have you ever stared at a tangled web of code, feeling like you’re trying to untangle a Gordian knot? Do you struggle with creating software that’s both robust and flexible? If so, you’re not alone. Software development is a complex endeavor, and finding the right solutions to recurring problems can be challenging.

Image: avelonpang.medium.com
Fortunately, there’s a treasure trove of knowledge available to help us navigate this intricate landscape: the Gang of Four design patterns. This collection of 23 tried-and-true solutions, elegantly documented in the influential book “Design Patterns: Elements of Reusable Object-Oriented Software”, provides a framework for addressing common design challenges in object-oriented programming. This article delves into the world of Gang of Four design patterns, exploring their history, their importance, and their practical applications. Buckle up, because you’re about to embark on a journey toward building better, more elegant software.
Deciphering the “Gang of Four”
The term “Gang of Four” refers to four authors — Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides — who revolutionized software design with their seminal work “Design Patterns.” Their book, often referred to as “GoF”, became the bible for object-oriented programmers, introducing a set of design patterns that have stood the test of time.
Why Design Patterns?
Design patterns offer a powerful solution to the recurrent challenges developers face when building complex software. They provide reusable solutions to recurring problems, promoting code reusability, flexibility, and maintainability.
Think of design patterns as blueprints for solving common design issues. They allow you to:
- Reduce redundancy by providing pre-built solutions to well-defined problems.
- Enhance code clarity through standardized and well-understood patterns.
- Improve flexibility by designing for adaptability and future modifications.
- Facilitate collaboration by establishing a common language among developers.
The Categories of Design Patterns
The Gang of Four categorized design patterns into three distinct groups based on their purpose and level of granularity:
- Creational Patterns: These patterns deal with object creation mechanisms, ensuring flexibility and control over the object instantiation process.
- Structural Patterns: These patterns focus on how objects are assembled into larger structures, addressing relationships and communication between objects.
- Behavioral Patterns: These patterns define how objects interact and collaborate with each other, focusing on how objects communicate and delegate responsibilities.
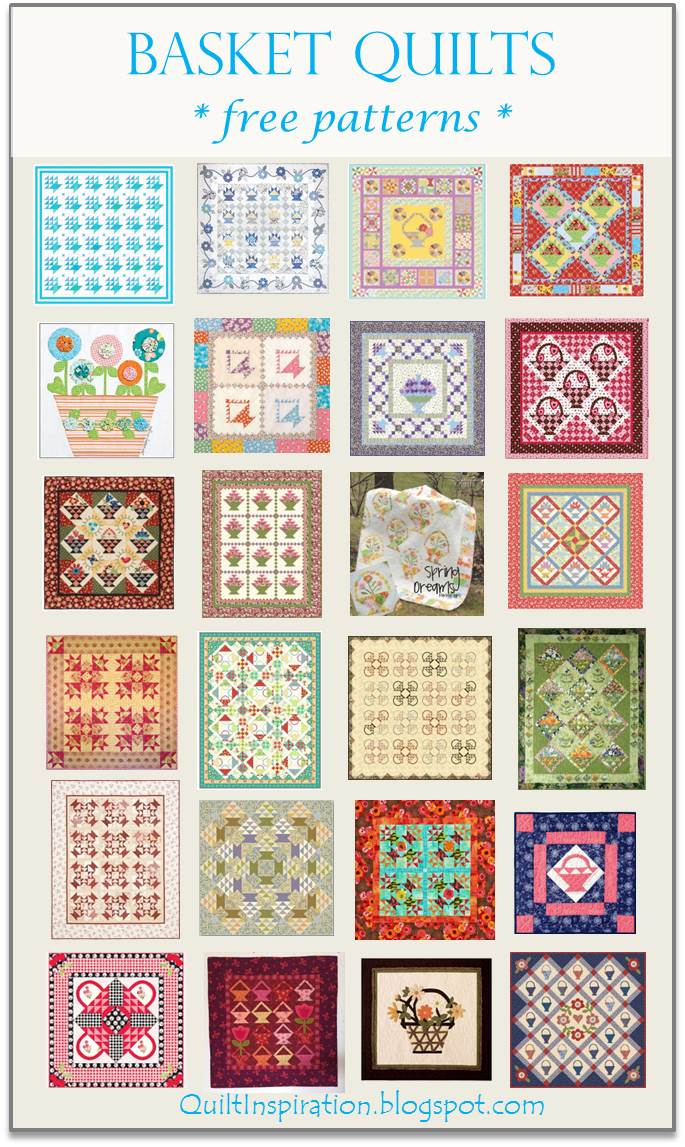
Image: guguaustin638.weebly.com
Exploring the Realms of Design Patterns
Creational Patterns: Shaping Your Objects
Creational patterns deal with the intricacies of object creation. Rather than directly instantiating objects, they use intermediaries to encapsulate the creation process, allowing for greater flexibility and customization. Let’s delve into the key creational patterns:
- Abstract Factory: A pattern for creating families of related objects without explicitly specifying their concrete classes. Think of it as a factory that manufactures different types of products, each with its own set of components (e.g., a furniture factory producing chairs, tables, and sofas).
- Builder: A pattern for constructing complex objects step-by-step. Think of it as a blueprint for building a house, allowing you to add rooms, walls, and fixtures sequentially.
- Factory Method: A pattern for creating objects without specifying their exact class. Instead, you define a common interface for creating objects and let subclasses determine the specific type to create. Imagine a factory that specializes in producing different types of vehicles — cars, motorcycles, or trucks — without explicitly defining the specific vehicle type at the factory level.
- Prototype: A pattern for creating new objects by cloning existing instances. This pattern is especially useful when you need to create objects with similar configurations, avoiding the need to re-specify all the attributes for each new instance.
- Singleton: A pattern for ensuring that a class has only one instance and provides a global point of access to that instance. This is often used for objects that need to be globally accessible and managed, such as a logger or a configuration manager.
Structural Patterns: Architecting Your Relationships
Structural patterns focus on how objects are organized and connected. They provide a blueprint for building robust and flexible structures that can adapt to changing needs. Here are some key structural patterns:
- Adapter: A pattern for converting the interface of a class into another interface that clients expect. Think of it as a translator bridging the communication gap between two incompatible systems. Imagine adapting a USB drive to connect to a laptop with only a Thunderbolt port.
- Bridge: A pattern for decoupling an abstraction from its implementation. It allows you to change the implementation without affecting the abstraction, promoting flexibility and modularity. Imagine separating the concept of a car’s engine (abstraction) from the actual engine implementation (implementation), allowing you to swap out different engine types.
- Composite: A pattern for composing objects into tree structures to represent part-whole hierarchies. Think of it as a recursive structure where each part can be either a single object or a group of objects. Imagine organizing a company hierarchy, with each manager being a composite of their direct reports.
- Decorator: A pattern for adding responsibilities dynamically to an object. Think of it as adding layers or decorations to an existing object, enhancing its functionality without altering its core structure. Imagine adding extra features like heated seats or a sunroof to a car after it’s been built.
- Facade: A pattern for providing a simplified interface to a complex subsystem. Think of it as a streamlined interface that hides the complexity of underlying components, making it easier for clients to interact with the subsystem.
- Flyweight: A pattern for sharing objects to support large numbers of fine-grained objects efficiently. Think of it as a pool of shared objects, where similar objects are reused instead of being repeatedly created. This is beneficial for resource-intensive operations involving a vast number of similar objects. Imagine sharing the same font object across multiple documents, instead of creating a separate font object for each document.
- Proxy: A pattern for providing a surrogate or placeholder for another object to control access to it. Think of it as a gatekeeper that intercepts requests and manages access to the underlying object. Imagine using a proxy server to control access to a website, acting as an intermediary between users and the website’s server.
Behavioral Patterns: Orchestrating Collaborations
Behavioral patterns focus on the interaction between objects and the communication between them. They provide a blueprint for defining how objects collaborate and communicate to achieve a common goal. Here are some key behavioral patterns:
- Chain of Responsibility: A pattern for avoiding tight coupling between the sender of a request and its receiver. It passes a request along a chain of handlers, giving each handler a chance to process the request. Imagine a chain of supervisors, each responsible for handling a specific type of request, with the request being passed along the chain until a handler can process it.
- Command: A pattern for encapsulating a request as an object. Think of it as a command object that stores information about a specific request, such as the receiver, the action to be performed, and any necessary parameters. Think of it like a button on a remote control that sends a command to the TV.
- Interpreter: A pattern for defining a grammatical representation for a language and providing an interpreter to deal with this grammar. Think of it as a translator for a specific language, enabling you to understand and execute commands in that language. Imagine building a compiler that understands a specific programming language and translates it into machine code.
- Iterator: A pattern for providing a way to access the elements of an aggregate object sequentially, without exposing its underlying representation. Think of it as a pointer that iterates through a collection of elements, allowing you to access each element without knowing the details of the collection’s implementation. Imagine using an iterator to traverse a list of items, one element at a time.
- Mediator: A pattern for defining an object that encapsulates how a set of objects interact. Think of it as a central coordinator that facilitates communication between multiple objects, avoiding direct interaction between them. Imagining a central traffic controller managing the flow of traffic at a busy intersection, ensuring that vehicles from different directions don’t collide.
- Memento: A pattern for capturing and externalizing an object’s internal state. Think of it as taking a snapshot of an object’s state, allowing you to restore it to a previous state at a later time. Imagine saving a document, capturing its current state, so that you can revert to that state if needed.
- Observer: A pattern for defining a one-to-many dependency between objects, where a change to one object notifies all its dependents. Think of it as a subscription service where observers are notified whenever the subject object changes. Imagine subscribing to email notifications whenever a specific product goes on sale.
- State: A pattern for allowing an object to alter its behavior when its internal state changes. Think of it as having multiple states, with different behavior associated with each state. Imagine a traffic light with different states (red, yellow, green) with different actions associated with each state.
- Strategy: A pattern for defining a family of algorithms, encapsulating each one and making them interchangeable. Think of it as a set of interchangeable strategies that can be selected and applied based on the specific context. Imagine different payment strategies (credit card, PayPal, bank transfer), each encapsulating a different payment method.
- Template Method: A pattern for defining the skeleton of an algorithm in a method, deferring some steps to subclasses. Think of it as a blueprint for an algorithm, with specific steps to be implemented by subclasses. Imagine creating a generic recipe for baking a cake, with specific steps that can be overridden by different cake recipes.
- Visitor: A pattern for representing an operation to be performed on the elements of an object structure. Think of it as a visitor that traverses an object structure and performs specific actions on each element. Imagine a visitor that checks the health of all the components in a complex system, performing different actions for each type of component.
The Gang of Four Design Patterns PDF: Your Toolkit
While the concepts behind design patterns are powerful, having readily available resources is crucial for their practical application. That’s where the Gang of Four design patterns PDF comes in. These PDFs provide a comprehensive and easily accessible guide to the world of design patterns.
These PDFs offer clear explanations, real-world examples, and visual diagrams to help you understand the essence of each pattern. They are invaluable tools for:
- Learning & Understanding: A comprehensive reference point for learning about design patterns, their application, and their respective strengths and weaknesses.
- Code Implementation: A practical guide for implementing design patterns in your code, providing clear structures and examples for application.
- Team Collaboration: A common language and framework for discussions on design and implementation, promoting effective communication within development teams.
The Future of Design Patterns: Evolution in Action
While the Gang of Four’s seminal work laid the foundation for design patterns, it’s important to recognize that the world of software development is constantly evolving. New languages, frameworks, and paradigms emerge, and design patterns need to adapt to these changes. We see a growing emphasis on:
- Domain-Specific Design Patterns: Tailored patterns that address specific domain challenges (e.g., cloud computing, mobile development, data science).
- Functional Programming Patterns: Design patterns adapted for functional programming paradigms, encouraging immutability, composition, and side-effect-free programming.
- Emerging Architectures: Design patterns adapted for microservices, event-driven architectures, and serverless computing models, addressing scalability and resilience.
- Artificial Intelligence and Machine Learning Patterns: Patterns emerging for architecting AI-driven systems, addressing challenges like data management, model training, and deployment.
Gang Of Four Design Patterns Pdf
Concluding Thoughts: The Value of Design Patterns
The Gang of Four design patterns are a foundational aspect of object-oriented software development. They provide a set of reusable solutions for common design challenges, promoting code reusability, flexibility, and maintainability.
While the original Gang of Four patterns provide a powerful foundation, the world of software development is constantly evolving. Exploring the latest advancements in design patterns, domain-specific patterns, and patterns adapted for emerging software architectures can further enhance your ability to create high-quality, adaptable, and maintainable software.
So, dive into the world of design patterns, explore the Gang of Four design patterns PDF, and embark on your journey towards building better software. You’ll be amazed at how these elegant solutions can transform your approach to software development.